In your line item descriptions, rather than using generic static text, you can create a function that defines a dynamic description that returns the specific options selected by the customer when they place an order.
New Order form
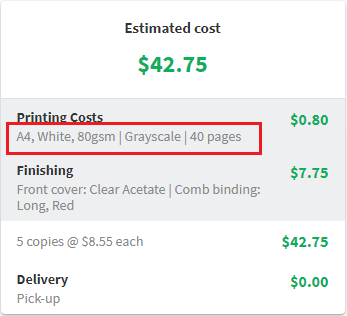
The New Order form, showing the order description.
This function is called a <line item>Description(order)
function, for example:
printingCostsDescription (order)
This function has the following structure:
- Define the function and pass in the order object
- Declare the variables
- Define the variables
- Define the description string
- Return a value back to the calling function
Example: Printing Costs description
New Order form
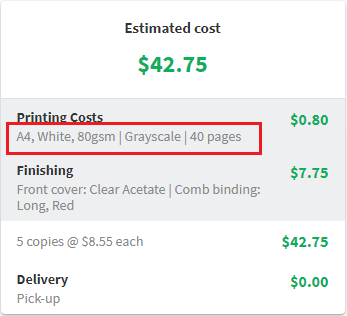
An exmaple of the New Order form, showing cost estimations.
Costing script
To create a dynamic description for a Printing Costs line item, add the following to your script:
Define the function and pass in the order object
function printingDescription(order) {
Declare the variables
var paperStockText;
var colorText;
var printingCostsText;
Define the variables
// 3.1 Concatenate the paper stock options
paperStockText = order.paperStock.size + ", " + order.paperStock.color + ", " + order.paperStock.type;
// 3.2 Determine whether to display color or grayscale
if (order.color.enabled) {
colorText = "Color";
} else {
colorText = "Grayscale";
}
Define the Printing Costs description string
printingCostsText = paperStockText + " | " + colorText + " | " + numberOfPages + " pages";
Return the value to the calling function
return printingCostsText
}
Example Finishing Costs description function
New Order form
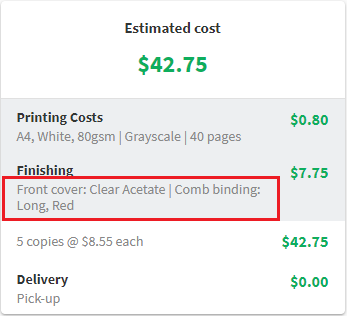
An exmaple of the New Order form, showing cost estimations.
Costing script
For Finishing options, the variable definitions are a bit more complex as Binding options can have sub-attributes.
Define the function and pass in the order object
function finishingDescription(order) {
Declare the variables
var frontCoverText = '';
var backCoverText = '';
var bindingText = '';
var bindingAttributeName = '';
var bindingSubAttributeText = '';
var finishingText = '';
Define the variables
// 3.1 Front cover description text (e.g. "Front Cover: Clear Acetate")
if (order.frontCover.name != 'No Cover') {
frontCoverText = "Front cover: " + order.frontCover.name +
(order.frontCover.printOnCover == null ? '' : ", " + order.frontCover.printOnCover)
}
// 3.2 Back cover description text(e.g. "Back cover: Frosted Acetate")
if (order.backCover.name != 'No Cover') {
backCoverText = "Back cover: " + order.backCover.name;
}
// 3.3 Binding descriptive text — Display the binding option only if it IS NOT 'No binding'
if (order.binding.name != 'No binding') {
bindingText = order.binding.name;
}
// 3.4 Binding descriptive text — Check if the selected binding option
// has sub-attributes (e.g. Position > Top left)
if (order.binding.attributes) {
// Display Comb Binding sub-attributes and the selected
// options (i.e. Binding Edge and Color).
// If 'Comb binding' is selected, this will produce text
// similar to: Comb binding: Long, Red.
if (order.binding.name == 'Comb binding'); {
bindingSubAttributeText = ":
" + order.binding.attributes[0].option.name
+ ", " + order.binding.attributes[1].option.name + " | "
}
// If 'Stapling' is selected, this will produce text similar:
// to Stapling: Top Left.
if (order.binding.name == 'Stapling') {
bindingSubAttributeText = ": " +
order.binding.attributes[0].option.name + " | "
}
}
Define the Finishing Costs description string
finishingText = frontCoverText + " | " + backCoverText + " | " + bindingText + bindingSubAttributeText;
// Remove any trailing vertical pipes in the description.
if (finishingText.endsWith('| '))
{
finishingText = finishingText.substring
(0, finishingText.length - 3);
}
Return the value to the calling function
return finishingText;
}
Comments